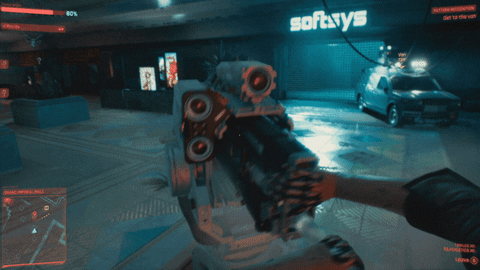
Beyond the 2D Plane: Creating Immersive Games with Three.js
In the realm of web development, Three.js stands out as a powerful JavaScript library for crafting stunning 3D graphics directly in the browser. While often associated with interactive visualizations and artistic projects, Three.js is also a fantastic tool for developing engaging and visually impressive 3D games.
Why Choose Three.js for Game Development?
While dedicated game engines like Unity and Unreal Engine offer comprehensive features, Three.js provides a compelling alternative for web-based 3D games, offering several key advantages:
- Web-Native and Accessible: Three.js games run directly in web browsers, eliminating the need for downloads or plugins. This inherent accessibility ensures a broad audience can experience your creations.
- JavaScript Familiarity: If you're already comfortable with JavaScript, Three.js provides a relatively smooth learning curve. You can leverage your existing skills to dive into 3D game development.
- Flexibility and Control: Three.js provides a low-level API, granting you fine-grained control over rendering, scene management, and object manipulation. This flexibility allows for highly customized game experiences.
- Open Source and Thriving Community: Being an open-source library, Three.js benefits from a vibrant community of developers constantly contributing and providing support.
- Lightweight and Performant: Three.js is designed for performance in the browser. While complex scenes require optimization, it's generally more lightweight than full-fledged game engines.
Core Concepts of Three.js for Games
To create games with Three.js, understanding these core concepts is essential:
- Scene: The foundation of your game. It's the container for all the objects, lights, and cameras in your 3D world.
- Camera: Defines the viewpoint from which the player sees the game world. Different camera types (perspective, orthographic) offer unique visual perspectives.
- Renderer: Responsible for drawing the scene onto the screen. Three.js supports various renderers, including WebGLRenderer (for hardware acceleration) and CSS3DRenderer (for leveraging CSS for 3D elements).
- Geometry: Defines the shape of 3D objects, such as cubes, spheres, and custom meshes.
- Material: Determines the visual properties of objects, such as color, texture, and reflectivity.
- Lights: Illuminate the scene, adding depth and realism. Three.js offers various light types, including ambient lights, directional lights, and point lights.
- Meshes: Combine geometry and material to create visible 3D objects in the scene.
- Game Loop: The heart of any game, the game loop continuously updates the game state (e.g., object positions, user input) and re-renders the scene.
- Animation: Animating objects using JavaScript to change object properties frame by frame to create movement and other dynamic effects.
- Physics Engines: For more realistic game behavior, integrating a physics engine (like Cannon.js or Ammo.js) allows for collision detection, gravity, and other physical interactions.
Building a Basic Three.js Game: A Simplified Example
While a full game is beyond the scope of this post, here's a simplified example showcasing the basic structure:
<!DOCTYPE html> <html> <head> <title>My Three.js Game</title> <style> body { margin: 0; overflow: hidden; } </style> </head> <body> <script src="https://threejs.org/build/three.js"></script> <script> // 1. Create the scene, camera, and renderer const scene = new THREE.Scene(); const camera = new THREE.PerspectiveCamera( 75, window.innerWidth / window.innerHeight, 0.1, 1000, ); const renderer = new THREE.WebGLRenderer(); renderer.setSize(window.innerWidth, window.innerHeight); document.body.appendChild(renderer.domElement); // 2. Create a cube const geometry = new THREE.BoxGeometry(); const material = new THREE.MeshBasicMaterial({ color: 0x00ff00 }); const cube = new THREE.Mesh(geometry, material); scene.add(cube); camera.position.z = 5; // 3. The game loop function animate() { requestAnimationFrame(animate); cube.rotation.x += 0.01; cube.rotation.y += 0.01; renderer.render(scene, camera); } animate(); </script> </body> </html>
This code creates a simple rotating cube. A real game would involve much more complexity, including user input handling, collision detection, game logic, and more sophisticated graphics.
Popular Three.js Game Examples
While Three.js might not be as common as other engines for large-scale games, it is still used:
- Roll it: A rolling ball game
- Sailing Simulators: Simple sailing simulations that leverage realistic physics.
Tips for Success with Three.js Game Development
- Start Small: Begin with simple projects to grasp the fundamentals before tackling more complex games.
- Optimize Performance: 3D rendering can be demanding. Optimize your code, reduce polygon counts, and use efficient textures to maintain smooth frame rates.
- Leverage Existing Libraries: Utilize libraries for physics (Cannon.js, Ammo.js), input handling, and other common game development tasks.
- Learn About Shaders: Shaders allow you to customize the rendering process, creating unique visual effects and improving performance.
- Study Examples: Explore open-source Three.js projects and examples to learn from experienced developers.
- Join the Community: Engage with the Three.js community for support, advice, and inspiration.
The Future of Three.js Games
As web technologies continue to advance, Three.js is poised to play an increasingly important role in web-based 3D gaming. With its accessibility, flexibility, and growing community, Three.js empowers developers to create immersive and engaging gaming experiences directly within the browser. So, if you're looking to venture into the world of 3D game development on the web, Three.js is definitely worth exploring.